Introduction
APIs (Application Programming Interfaces) have become ubiquitous in modern software development. They allow different applications to communicate with each other by exposing functionality and data. With APIs, developers don’t have to reinvent the wheel each time they need some common functionality.
The architecture of an API determines how it is designed and built. Various architectural styles have emerged over the years, each with their own benefits and drawbacks. Choosing the right style can have significant impacts on an API’s flexibility, performance, and ability to evolve.
In this article, we’ll explore the 6 most popular API architecture styles and when you may want to use each one.
Overview of API Architecture Styles
Here is a quick overview of the API architecture styles we’ll cover:
Style | Description |
---|---|
REST | Uses HTTP methods to expose resources. Responses usually JSON or XML. |
GraphQL | Allows clients to declaratively fetch only needed data. Single endpoint. |
RPC | Uses request/response model with remote function calls. Often uses protocols like gRPC. |
Webhook | Registers callbacks on server. Triggers callback when event occurs. |
Async | Uses messaging systems like queues to enable async communication. |
Event-driven | API events trigger compute, storage, downstream APIs, etc. |
Let’s explore each of these in more detail.
REST (Representational State Transfer)
REST (Representational State Transfer) is arguably the most common API architecture style. It leverages HTTP protocols and methods to expose resources. Some key principles of REST include:
- Exposing resources with unique URIs (like
/users/123
) - Use HTTP methods to manipulate resources (GET, POST, PUT, DELETE, etc)
- Stateless – no client session data stored on server
- Responses usually JSON or XML formatting
REST APIs aim to expose data entities and actions on those entities. For example, you may have endpoints like:
- GET /users – get a list of users
- GET /users/123 – get details about user 123
- POST /users – create a new user
- PUT /users/123 – update user 123
- DELETE /users/123 – delete user 123
REST APIs tend to be simple, lightweight, and easy to build and consume. This makes them a good default choice for many API scenarios, especially when exposing CRUD functionality.

Some downsides are that REST APIs can sometimes result in over-fetching data and can require multiple round trips to assemble needed data. They also provide limited ways to notify clients of changes or events.
Good use cases include:
- Public APIs needing wide consumption
- APIs focused on CRUD functionality
- Simple APIs without much real-time or event handling needs
GraphQL
GraphQL is a newer api architecture styles that provides a declarative alternative to REST. With GraphQL, the client specifies exactly what data it needs via a query language. Some key aspects:

- Strong typing – queries and responses have a well-defined structure
- Hierarchical – related data can be nested for efficiency
- Declarative – client specifies what it needs, not how to get it
- Single endpoint – all requests go to one URL
For example, a query may look like:
query {
user(id: "123") {
name
age
address {
street
city
}
}
}
This allows the client to retrieve exactly the fields it needs in a single round trip. The shape of the response matches the query structure.
GraphQL APIs provide flexibility, prevent over-fetching, and allow efficient data access. The major downside is increased complexity on the server to handle the flexibility of queries.
Good use cases include:
- Apps that need to fetch nested, relational data
- Apps that need flexibility to ask for different data
- Situations where you want to prevent over-fetching
RPC (Remote Procedure Call)
Remote Procedure Call(RPC)-based APIs expose services and procedures that can be invoked remotely. Clients make calls as if invoking a local function, but it executes on the server. Some examples:
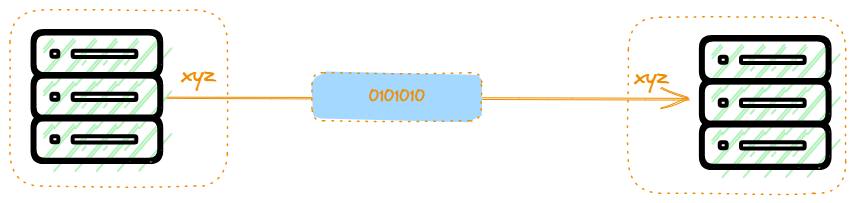
- gRPC – uses protocol buffers and proto files to define services
- JSON-RPC – similar but uses JSON for requests/responses
- XML-RPC – uses XML for data formats
RPC APIs commonly have these traits:
- Request/response model – client makes request, server returns response
- Strongly typed – payloads and API interfaces strictly defined
- Use IDL (Interface Definition Language) to define services
- Can auto-generate client libraries from IDL
RPC APIs allow you to expose functionality in a language-agnostic way with static typing. However, they are not as loosely coupled since clients and servers need to agree on interfaces.
Good use cases include:
- APIs tightly coupled to an application
- Efficient request/response needs
- Remote function calls between services
- Situations where you want static typing
Webhook
Webhooks provide a way to notify clients when events occur. Clients register callback URLs with the API, then the API triggers these callbacks when something happens. Some key aspects:

- Event-driven – API invokes callbacks on events
- Loose coupling – clients and API interact asynchronously
- Often uses HTTP POST to callback URLs
- Payloads contain event data
For example, a client may register this webhook URL:
https://example.com/api/handleNewUser
When a new user is created, the API would POST event data to that URL.
Webhooks allow you to build reactive, event-driven systems. Downsides are added complexity handling callbacks and no standard webhook protocol.
Good use cases include:
- Notifying external systems about events
- Integrating or chaining APIs together
- Building reactive flows
- Situations where you need loose coupling
Async Messaging
Asynchronous messaging architectures use a message broker or queue to facilitate communication between the client and API. Examples include RabbitMQ, Kafka, etc. Some key aspects are:
- Loose coupling – clients and API interact asynchronously
- Queue-based – requests go on queue, responses put on another queue
- Can support various patterns likeCompeting Consumers, CQRS, etc.
- Adds reliability and scalability for high-load
In this style, clients would put requests on a “requests” queue. The API servers would consume these requests asynchronously. They would then put responses onto a separate “responses” queue that clients read from.
Async messaging adds reliability and scalability by decoupling clients from servers. It does add complexity of messaging infrastructure.
Good use cases:
- High-load APIs needing scalability
- Situations where loose coupling is beneficial
- Interacting with legacy systems
- Facilitating complex workflows and queues
Event-Driven Architecture
In an event-driven architecture, the API exposes and reacts to events. Clients can emit events, while the API uses them to trigger additional compute, storage, downstream API calls, etc. Some key aspects:
- Event producers / consumers – clients emit, API reacts
- Reactive – API triggered by event data
- Promotes decoupling – clients emit events independently
For example, a client may emit an UserSignedUp
event containing new user details. The API would react to this by storing to a database, sending a welcome email, updating related systems, etc.
This allows you to build reactive, event-driven workflows. It does add complexity of handling events and hooking into reaction logic.
Good use cases:
- Building reactive / event-driven systems
- Decoupling subsystems that can react independently
- API driving complex workflows
- Situations where you want to offload work
Summary Table of the API architecture styles
Here is a summary of the API architecture styles:
Style | Description | Benefits | Drawbacks | Use Cases |
---|---|---|---|---|
REST | Resources, HTTP methods | Simple, lightweight, scalable | Over-fetching, many round trips | Public APIs, CRUD |
GraphQL | Declarative, strongly typed | Flexible queries, efficient | Complexity on server | Apps needing flexibility |
RPC | Request/response, remote functions | Language agnostic, strongly typed | Tight coupling | Internal APIs, microservices |
Webhook | Event callbacks | Loose coupling, reactive | Complex delivery handling | Event notifications, chaining APIs |
Async Messaging | Queue-based communication | Reliable, scalable, decoupled | Added infrastructure complexity | High-load APIs, workflow automation |
Event-Driven | API reacts to event data | Reactive, decoupled | Complex event processing | Reactive systems, workflows |
FAQ
What is the most common API architecture style?
The most common and ubiquitous API architecture style is REST (Representational State Transfer). Its simplicity and wide adoption make it a good default choice for many API use cases, especially when doing basic CRUD operations.
Should I use GraphQL or REST for my API?
GraphQL is great when you need to efficiently retrieve nested, relational data in fewer round trips. It provides more flexibility for clients. REST is simpler and good for basic CRUD APIs accessed by many types of clients. Evaluate both and consider the tradeoffs for your specific needs.
Is RPC better for performance than REST?
Not necessarily. Well-designed REST APIs can provide excellent performance by leveraging HTTP caching, good database schemas, and other optimizations. However, RPC can reduce chattiness in some scenarios by allowing one request to retrieve multiple related bits of data efficiently. Evaluate performance requirements and consider tradeoffs.
When would I use asynchronous messaging or event-driven architectures?
These styles promote loose coupling and reactivity by using queues or events. Useful in situations like workflow automation, integrating with legacy systems, or offloading work from clients. Adds complexity so only use if you need these benefits. Simpler styles like REST suffice for many common API scenarios.
Can different architectures be combined?
Absolutely. For example, you could expose a REST API but use asynchronous queuing internally to promote loose coupling and reliability. Or, register webhooks from a REST API to add reactive event notifications. Mix and match architectural styles based on your specific needs.
Conclusion
There are a variety of API architecture styles, each with their own strengths and tradeoffs. Considering factors like performance, flexibility, complexity, and coupling will help you choose the right approach. Many successful APIs use a combination of architectural styles where it makes sense. API architecture styles has a major impact on usability, so choose wisely based on your specific use case.