Introduction
Node.js is a popular runtime for fast web applications thanks to its asynchronous event-driven architecture. But Scaling Node.js applications pose challenges for beginners when moving to production.
In this guide, you’ll learn how Docker and Kubernetes provide simple yet powerful solutions for taking Node.js apps to the next level – enabling smooth scaling, high availability and simplified deployment.
We’ll walk through containerizing Node.js apps with Docker, managing multiple containers with Kubernetes, and best practices for optimizing and monitoring Node.js in production. Let’s get started scaling Node.js Applications.
Why Scale Node.js Apps?
Node.js apps are fast – but suffer scaling bottlenecks like:
- Blocking I/O calls degrade parallelism
- Single-threaded design limits compute scaling
- Stateless architecture complicates session management
- Package dependencies and versions must be consistent
Thankfully, modern infrastructure provides great solutions. Specifically, Docker and Kubernetes help Node.js apps:
- Achieve high availability – Mitigate downtime through redundancy
- Improve reliability – Limit crashes and errors through replication
- Scale seamlessly – Spin nodes up and down to handle load changes
- Standardize environments – Helps avoid “works on my machine” woes
- Streamline deploys – Replace old versions easily through automation
Docker and Kubernetes provide beginner-friendly ways to address Node’s scaling pain points.
Scaling Node.js Applications with Docker and Kubernetes
Dockerizing Node.js Apps
Docker is the de facto standard for packaging apps in containers. For Node.js, Docker provides:
- Consistent environments – Dependencies and versions locked in
- Isolation – Apps separated from infrastructure
- Portability – Easily run apps anywhere
- Microservices – Decompose into modular containers
- Standardization – Using best practices for container builds
With Docker, Node.js apps can reliably run from dev, test, all the way through production.
Creating a Dockerfile
A Dockerfile describes how to package a Node app as a Docker image:
FROM node:16-alpine
WORKDIR /app
COPY . .
RUN npm install
CMD ["node", "server.js"]
This pulls a Node.js base image, adds the app, installs dependencies, and specifies the runtime command – resulting in a containerized Node.js application.
Build the container image using docker build
.
Running Containers
Next we can run the containerized app:
docker run -p 3000:3000 my_node_app
This exposes port 3000 externally while running Node inside the container.
Docker enables portable Node.js apps abstracted from infrastructure.
Orchestrating Containers with Kubernetes
While Docker packages apps as containers, Kubernetes helps manage and scale those containers across multiple servers.
Kubernetes gives:
- High availability – Spread containers across nodes so failures don’t disrupt
- Load balancing – Distribute traffic evenly using Kubernetes Services
- Zero downtime deploys – Replace containers without interruption
- Autoscaling – Automatically spin up more containers to handle traffic spikes
- Secret management – Store configs and credentials securely
- Monitoring – Insight into container performance and health
With Kubernetes orchestrating containers, Node.js apps gain enterprise-grade availability, scalability and operability.
Basic Kubernetes Architecture
Kubernetes follows a master-worker architecture:
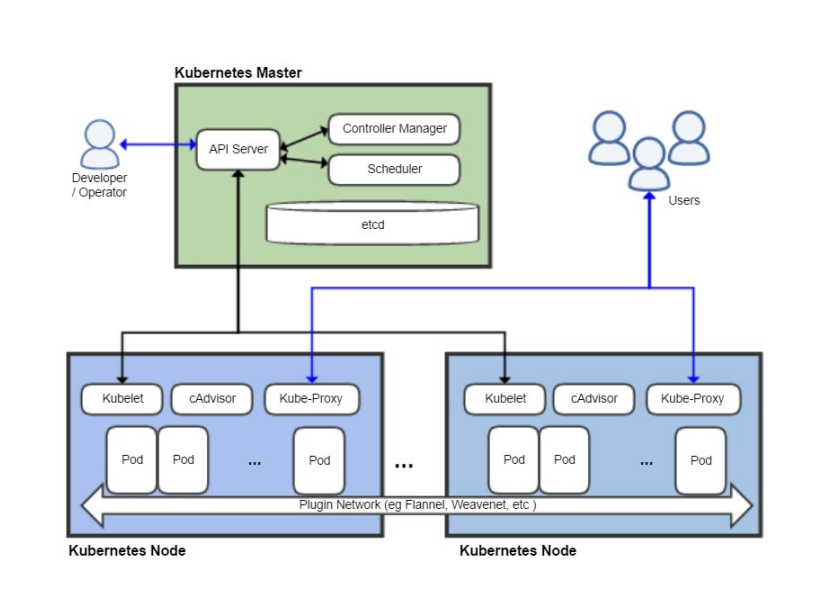
The Kubernetes master manages a group of nodes running containerized applications.
We can deploy apps by defining Kubernetes resources like Deployments and Services in config files.
Running a Node.js App on Kubernetes
To run a Node app on Kubernetes:
- Containerize the Node.js app with a Dockerfile
- Build a Docker image from the Dockerfile
- Push the Docker image to a registry like Docker Hub
- Define a Deployment manifest for the Node.js app
- Create the Deployment on the Kubernetes cluster
- Expose the Deployment through a Service
Once running, Kubernetes provides discovery between containers and load balancing to scale Node.js apps.
Optimizing Node.js App Performance
To maximize Node performance, consider:
- Increase throughput – Use worker threads to handle blocking I/O outside event loop
- Scale horizontally – Run multiple Node.js container instances managed by Kubernetes
- Use faster runtimes – Try Node interpreters like Fastify for speed gains
- Reduce payload sizes – Minify responses, enable compression
- Tune garbage collector – Increase memory limits, adjust GC flags
- Analyze bottlenecks – Profile with Artillery, cAdvisor
- Add caching – Redis, CDNs to reduce database loads
Optimizing Node.js for production is key to reduce latency and costs.
Monitoring Node.js Apps
Robust monitoring provides visibility into production Node.js apps:
- App metrics – Capture KPIs like request rates, response times, error counts
- Distributed tracing – Follow requests across services, identify hot spots
- Log aggregation – Collect and analyze logs in tools like the ELK stack
- Alerting – Get notified for critical failures and performance changes
- APM tools – End-to-end app performance monitoring and troubleshooting
Monitor across layers – from containers to hardware – to manage Node.js apps at scale.
Conclusion
Node.js provides fast and efficient web apps, but requires expertise to scale smoothly. With Docker and Kubernetes:
- Docker containers enable building and deploying Node.js apps to any environment
- Kubernetes handles coordinating containers across servers and scaling needs
- Optimizations and monitoring deliver high-performance production Node.js apps
By leveraging containers and orchestration, you can run Node.js apps reliably at any scale. Docker and Kubernetes give beginners simple yet enterprise-grade solutions for taking Node.js apps to the next level.
Frequently Asked Questions
Q: Can Docker and Kubernetes help legacy Node.js apps scale?
A: Absolutely – you can “lift and shift” Node.js apps into Docker and Kubernetes to add portability and orchestration without code changes.
Q: Is Kubernetes overkill for simple Node.js apps?
A: Potentially – evaluate complexity versus benefits. But Kubernetes can run small Node.js apps efficiently as well.
Q: How do Docker and Kubernetes compare to hosting Node.js on VMs?
A: Containers provide greater portability and efficiency. But VMs may still have a place, for example to isolate teams.
Q: What other tools work well with Node.js, Docker and Kubernetes?
A: Helm for package management, Prometheus for monitoring, Grafana for visualization, Envoy for load balancing, gRPC for communication.
Q: Where can I learn more about production best practices with Node.js?
A: The Node.js Production Checklist, Node.js Production Best Practices book, and Node.js docs on containers provide great information.